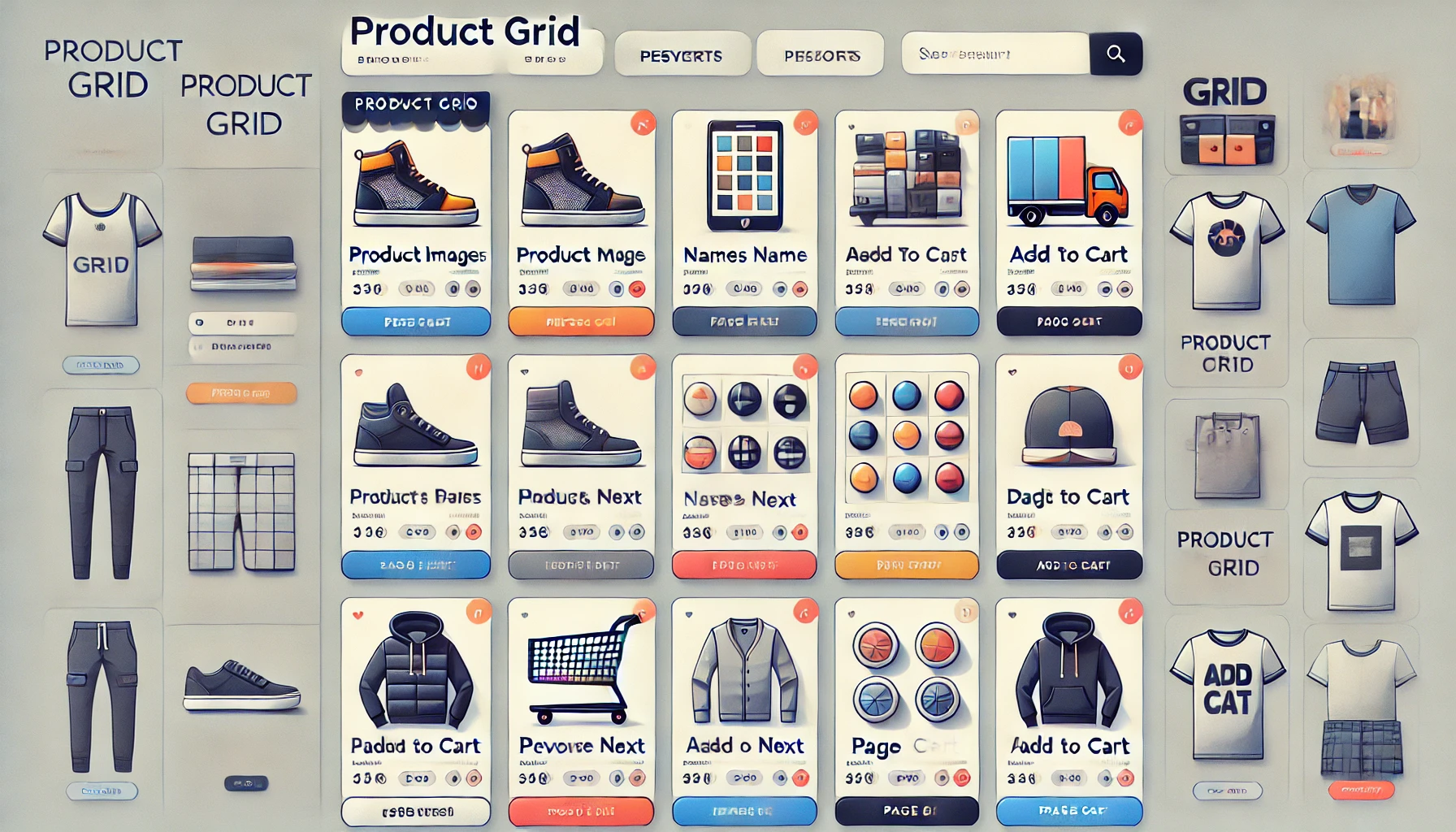
Bootstrap product grid with pagination, using PHP’s foreach loop to dynamically load products. It displays products in a clean and organized layout with pagination controls at the bottom.
# Bootstrap Product Grid with Paging Using Foreach in PHP
<?php
// Sample data array
$products = [
['id' => 1, 'name' => 'Product 1', 'price' => 10.00],
['id' => 2, 'name' => 'Product 2', 'price' => 15.00],
['id' => 3, 'name' => 'Product 3', 'price' => 20.00],
['id' => 4, 'name' => 'Product 4', 'price' => 25.00],
['id' => 5, 'name' => 'Product 5', 'price' => 30.00],
['id' => 6, 'name' => 'Product 6', 'price' => 35.00],
['id' => 7, 'name' => 'Product 7', 'price' => 40.00],
['id' => 8, 'name' => 'Product 8', 'price' => 45.00],
['id' => 9, 'name' => 'Product 9', 'price' => 50.00],
['id' => 10, 'name' => 'Product 10', 'price' => 55.00],
];
// Pagination variables
$itemsPerPage = 5;
$totalItems = count($products);
$totalPages = ceil($totalItems / $itemsPerPage);
$currentPage = isset($_GET['page']) ? (int)$_GET['page'] : 1;
$offset = ($currentPage - 1) * $itemsPerPage;
// Slice the products array for the current page
$currentProducts = array_slice($products, $offset, $itemsPerPage);
?>
<div class="container">
<div class="row">
<?php foreach ($currentProducts as $product): ?>
<div class="col-md-3">
<div class="card">
<div class="card-body">
<h5 class="card-title"><?php echo $product['name']; ?></h5>
<p class="card-text">$<?php echo number_format($product['price'], 2); ?></p>
<a href="#" class="btn btn-primary">View Product</a>
</div>
</div>
</div>
<?php endforeach; ?>
</div>
<nav aria-label="Page navigation">
<ul class="pagination">
<?php for ($i = 1; $i <= $totalPages; $i++): ?>
<li class="page-item <?php echo $i === $currentPage ? 'active current' : ''; ?>">
<a class="page-link" href="?page=<?php echo $i; ?>"><?php echo $i; ?></a>
</li>
<?php endfor; ?>
</ul>
</nav>
</div>
Demo – https://a2zeshop.com/products/
Hire freelancer – https://www.ordermanagersystem.com/#Contact